In the ever-evolving landscape of software development, ensuring the delivery of high-quality, robust code remains a paramount concern. Test-driven development (TDD), a methodical and disciplined approach, emerges as a cornerstone in achieving this goal. This blog delves into the essence of TDD, a technique that not only revolutionizes the way developers write code but also elevates the overall quality and reliability of software products. By prioritizing tests before coding, TDD fosters a culture of meticulous planning, anticipates potential issues, and paves the way for a more streamlined and efficient development process. Here, we unpack the fundamentals of TDD its profound impact on code quality, and demonstrate its application through a practical example in Java.
Test-driven development (TDD) can be likened to an architect constructing a building by first creating a detailed scale model. Just as an architect meticulously crafts a model to visualize and test the structure, functionality, and aesthetics of a building before laying the first brick, TDD requires developers to write and test a precise, miniature version of their code (the test) before developing the actual software. This approach ensures that every aspect of the software is planned, tested, and validated in advance, much like an architect's model guides and informs the construction process, leading to a well-structured, stable, and functional final product. In both cases, the initial model or test acts as a blueprint, setting a clear path and expectations, which significantly reduces the risk of errors and ensures a high-quality outcome.
Test-driven development (TDD) is a software development process that encourages a disciplined approach to software development. It promotes better code design and structure, facilitates early detection and correction of defects, improves code maintainability and extensibility, and provides a safety net for refactoring and code changes.TDD starts with writing a test that defines the expected behavior of a piece of code before the code itself is written. This forces developers to think about the requirements and design of the code before they start implementing it, leading to better code quality and design. By writing the test first, developers are forced to consider the expected behavior of the code and design it accordingly, rather than writing code and then trying to figure out how to test it. This approach helps to identify and fix problems early in the development process, reducing the cost of fixing defects later on.
TDD also promotes the use of refactoring, which is the process of improving the structure and design of code without changing its functionality. Because the tests provide a safety net, developers can refactor code without fear of breaking it, leading to more maintainable and extensible code.
In summary, TDD is a valuable practice that promotes better code design, early detection of defects, improved code maintainability and extensibility and supports refactoring. By writing tests first, developers can ensure that their code meets the requirements and is of high quality, leading to more reliable and maintainable software.
TDD Example
Let's take a simple example of Test-Driven Development (TDD) in Java. Suppose we want to create a function that adds two integers. We'll follow the TDD steps: write the test first, see it fail, write the minimum code to pass the test, and then refactor if necessary.
1) Write the Test First
We'll use JUnit for writing the test. Let's create a test class named CalculatorTest.
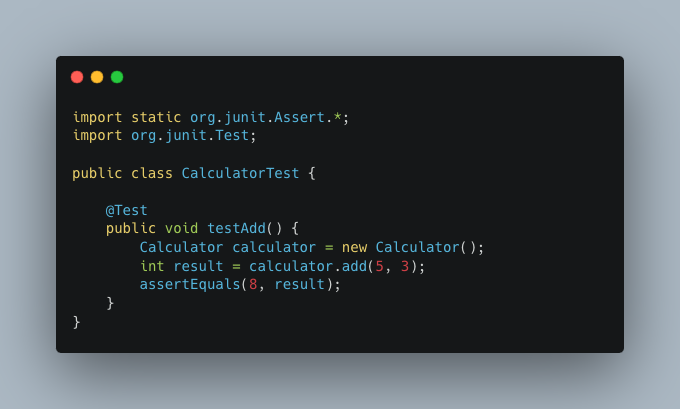
In this test, we're trying to use a Calculator class and its method add, which don't exist yet. So, this test will fail, which is expected in TDD.
2) See the Test Fail
Running this test at this stage should result in a compilation error since Calculator and its method add are not implemented yet.
3) Write Minimum Code to Pass the Test
Now, let's create the Calculator class with the minimum necessary code to pass the test.
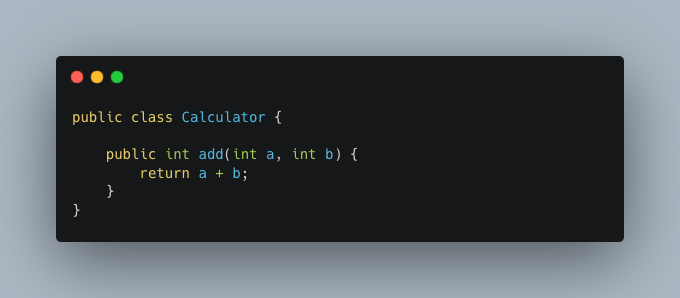
4) Run the Test and See it Pass
With this implementation, if we run the CalculatorTest, it should pass, as our add method correctly implements the required functionality.
5) Refactor if Necessary
At this point, we can refactor our code if needed. For this simple example, refactoring might not be necessary, but in more complex scenarios, this step would involve optimizing the code, improving readability, or considering edge cases.
TDD encourages writing tests that dictate and validate what the code will do. In real-world scenarios, TDD often involves more complex setups, consideration of edge cases, and continuous integration of new tests and code refinements.
Test-driven development stands out as a beacon of quality assurance in the software development process. By compelling developers to write tests first and code second, TDD inculcates a proactive mindset, ensuring that every piece of code is purpose-built to meet its intended functionality. The methodical cycle of writing tests, witnessing initial failures, coding to pass these tests, and then refining the code, exemplifies a rigorous approach to software development. As illustrated through the Java example, TDD is not just a theoretical concept but a practical and impactful tool, capable of transforming the quality, maintainability, and extensibility of code. Adopting TDD is more than a development strategy; it is a commitment to excellence, ensuring the creation of software products that are not only functional but also resilient and future-proof.