In the intricate world of software testing, the use of mock objects stands out as a vital technique for simplifying and streamlining the testing process. Much like a stunt double in a movie, mock objects take the place of real dependencies, allowing developers to focus on the specific aspects of the system they are testing. This blog explores the concept of mock objects, illustrating how they imitate the behavior of actual dependencies to isolate the system under test. By offering a controlled testing environment, mock objects significantly enhance the efficacy, flexibility, and speed of the testing process, proving indispensable in the realm of unit testing for Java applications.
Mock objects in software testing can be likened to crash test dummies used in car safety testing. Just as crash test dummies simulate the human body's response in a car crash, allowing engineers to evaluate the safety features of a vehicle without putting real humans at risk, mock objects simulate the behavior of real system dependencies. This simulation enables developers to test and refine their software's interactions and responses in a controlled, risk-free environment. The use of mock objects, like crash test dummies, ensures that the product (be it a car or a software application) can perform as expected in real-world scenarios without the complications and unpredictability of testing with actual human subjects or live system dependencies.
Mock objects: imitating dependencies for effective testing
Mock objects are objects that simulate the behavior of real objects in order to isolate the system under test from its dependencies. This can improve test isolation, verify interactions between the system under test and its dependencies, increase test flexibility, and enhance test performance. By simulating the behavior of real objects, mock objects allow developers to test specific components of a system without the need to create or interact with the actual dependencies. This can significantly reduce the complexity and time required for testing, as developers can focus on the behavior of the system under test without worrying about the behavior of its dependencies.
Mock objects can also be used to verify interactions between the system under test and its dependencies. By defining the expected interactions between the system under test and its dependencies, mock objects can help developers ensure that the system under test is behaving as expected. This can be especially useful when testing complex systems with many dependencies, as it allows developers to quickly and easily verify that the system is interacting with its dependencies correctly.
In addition, mock objects can increase test flexibility by allowing developers to easily change the behavior of dependencies without having to modify the system under test. This can be useful when testing different scenarios or conditions, as developers can quickly and easily configure the mock objects to simulate different behaviors.
Finally, mock objects can enhance test performance by reducing the overhead associated with creating and interacting with real dependencies. This can be especially beneficial when testing large or complex systems, as it can significantly reduce the time required to run tests.
Overall, mock objects are a powerful tool for unit testing Java applications. They can improve test isolation, verify interactions between the system under test and its dependencies, increase test flexibility, and enhance test performance.
Example
We'll use a common scenario: testing a service class that depends on a data access object (DAO). The mock object will simulate the behavior of the DAO.
First, ensure you have a testing framework and a mocking framework included in your project. For this example, we'll use JUnit for testing and Mockito for mocking.
Suppose we have a UserService class that depends on a UserDao class. The UserService has a method findUserByEmail that retrieves user details based on the email address.
Here's what the classes might look like:
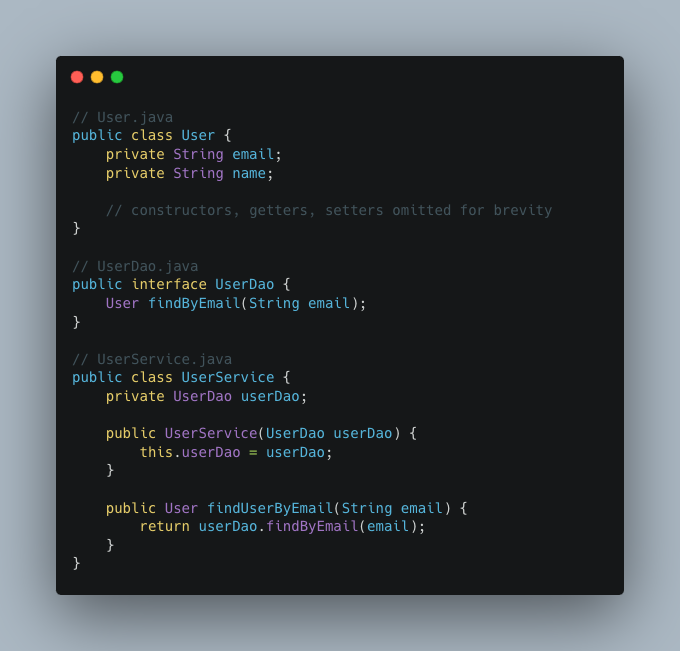
Now, let's write a test for the UserService class using a mock UserDao:
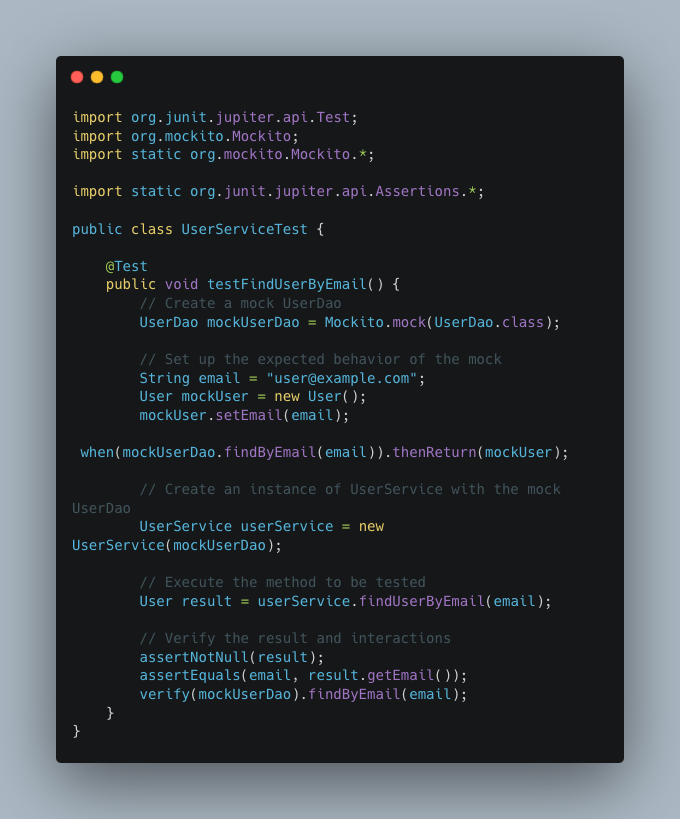
In this test, we're using Mockito to create a mock UserDao. We then specify the behavior of the findByEmail method when it's called with a specific email. This allows us to test the UserService without relying on the actual implementation of UserDao, thus isolating the test to only the functionality of UserService.
In summary, mock objects serve as a crucial component in the toolkit of modern software development, particularly in unit testing. They offer a streamlined, efficient approach to testing, akin to rehearsing a play with stand-ins for actors who are not available. By imitating real dependencies, mock objects simplify the testing landscape, allowing developers to conduct thorough and focused tests. This not only leads to more robust and reliable software but also accelerates the development cycle. The strategic use of mock objects, therefore, transcends mere testing techniques; it embodies a philosophy of precision and efficiency in software development, ensuring the creation of high-quality applications equipped to meet the challenges of a dynamic technological landscape.