In the realm of software testing, clarity and structure are key to ensuring that unit tests are both effective and easy to understand. The Arrange-Act-Assert pattern stands as a testament to this principle, offering a streamlined approach to organizing tests. This pattern, akin to a well-crafted recipe, breaks down the testing process into three distinct yet interconnected phases: Arrange, Act, and Assert. Each phase plays a crucial role in setting the stage for a successful test, executing the test, and verifying the outcomes. Let's review each of these phases and explore how they collectively contribute to the creation of robust and reliable unit tests.
The Arrange-Act-Assert pattern can be compared to a chef preparing a gourmet dish. In the Arrange phase, the chef gathers all necessary ingredients and equipment, much like a developer sets up the test environment and prepares the required objects and their states. The Act phase resembles the actual cooking process, where the chef skillfully combines and cooks the ingredients, analogous to the developer executing the method under test. Finally, the Assert phase is akin to the tasting session, where the chef verifies if the dish meets the expected flavor, texture, and presentation - similar to the developer checking if the test results align with the expected outcomes. This methodical approach in both cooking and testing ensures a consistent, high-quality result, be it a delightful dish or a bug-free software application.
Arrange-Act-Assert
The Arrange-Act-Assert pattern is a simple and effective way to structure unit tests. It consists of three phases: Arrange, Act, and Assert. In the Arrange phase, you set up the test fixture. This includes creating any objects that are needed for the test and initializing their state. For example, if you are testing a method that calculates the area of a circle, you would create a Circle object and set its radius to a specific value.
In the Act phase, you call the method that you are testing. In the example of the circle area calculation, you would call the method that calculates the area of the circle.
In the Assert phase, you verify that the method produced the expected results. In the example of the circle area calculation, you would assert that the area of the circle is equal to the expected value.
The Arrange-Act-Assert pattern is a valuable tool for writing effective and efficient unit tests. It helps to ensure that your tests are well-structured and easy to understand, and it makes it easier to identify and fix bugs in your code.
Example
Let's create an example to demonstrate the Arrange-Act-Assert pattern in the context of unit testing. We'll use a simple Java class and JUnit for testing.
Suppose we have a Calculator class with a method add that takes two integers and returns their sum. We will write a test for this method using the Arrange-Act-Assert pattern.
Here's the Calculator class:
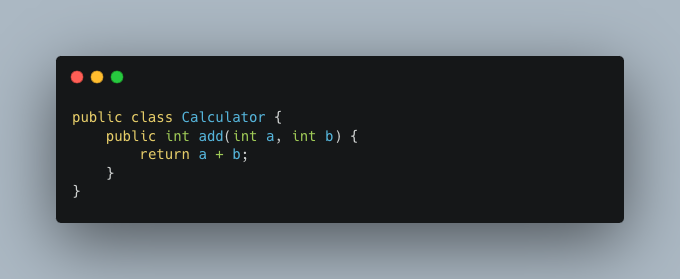
Now, let's write a unit test for the add method:
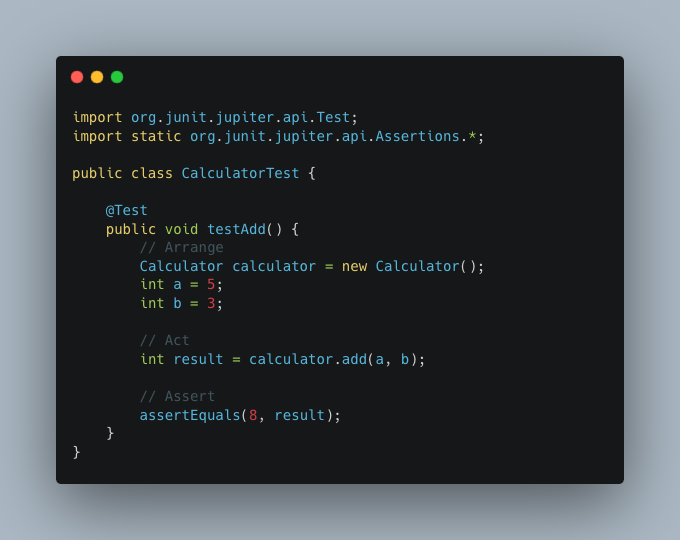
In this test:
- Arrange: We create an instance of Calculator and initialize the variables a and b with the values we want to add. This sets up the necessary state for the test.
- Act: We call the add method of the Calculator instance with the variables a and b. This is where the actual operation we want to test is performed.
- Assert: We use assertEquals to check if the result of the add method is as expected. In this case, we assert that the sum of 5 and 3 should be 8.
This test is structured clearly, making it easy to understand what is being set up, what is being tested, and what is being verified.
In conclusion, the Arrange-Act-Assert pattern is an invaluable framework in the world of software testing, providing a clear and methodical approach to crafting unit tests. By segmenting the test into distinct phases - setup, execution, and verification - this pattern not only simplifies the testing process but also enhances its efficacy. Just as a chef methodically prepares, cooks, and evaluates a dish, developers using the Arrange-Act-Assert pattern can meticulously prepare their tests, execute them with precision, and accurately assess the results. This not only leads to more reliable and maintainable code but also fosters a culture of thoroughness and precision in the software development process.